2020. 3. 18. 20:20ㆍ카테고리 없음
- Producer Consumer Problem In Java Using Wait And Notify
- Java Consumer Producer
- Producer Consumer Problem C++
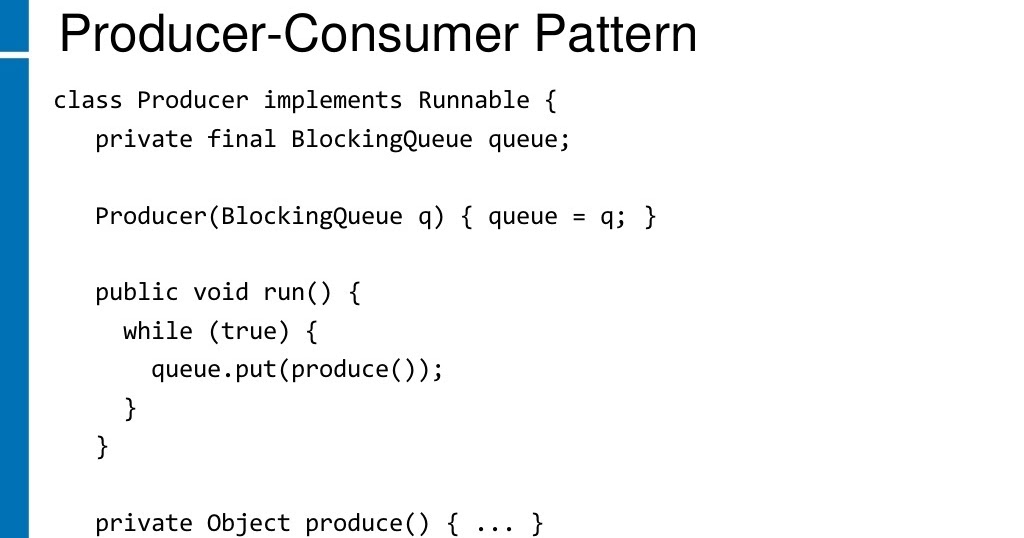
Producer Consumer problem is a classic concurrency problem and is often asked in interview. The problem goes as follows: Producer and Consumer are two separate threads which share a same bounded Queue. The role of producer to produce elements and push to the queue. The producer halts producing if the queue is full and resumes producing when the size of queue is not full. The consumer consumes the element from the queue. The consumers halt consuming if the size of queue is 0 (empty) and resumes consuming once the queue has an element.The problem can be approached using various techniques. Using wait and notifyAll.
Using sempahoresHow to use wait and notifyAll in codeSome of the best practices are listed belowwait and notifyAll are the fundamental method of the Object class. Solving this problem, helps understand some of the fundamentals of OOPs and concurrency.wait and notifyAll is shared on the same object between two threads. In this case it will be the Queue.wait and notifyAll should be used inside the synchronized block.The methods should be called inside the for loop.
Producer Consumer Problem In Java Using Wait And Notify
Since wait is called inside a conditional block e.g. Producer thread should call wait if queue is full, first instinct goes towards using if block, but calling wait inside if block can lead to subtle bugs because it's possible for thread to wake up spuriously even when waiting condition is not changed.
Is pretty complex topic and requires a lot of attention while writing application code dealing with multiple threads accessing one/more shared resources at any given time. Java 5, introduced some classes like and Executors which take away some of the complexity by providing easy to use APIs.Programmers using concurrency classes will feel a lot more confident than programmers directly handling synchronization stuff using wait, notify and notifyAll method calls.
I will also recommend to use these newer APIs over synchronization yourself, BUT many times we are required to do so for various reasons e.g. Maintaining legacy code.
A good knowledge around these methods will help you in such situation when arrived. In this tutorial, I am discussing the purpose of wait notify notifyall in Java. We will understand the difference between wait and notify.Read more: 1. What are wait, notify and notifyAll methods?The Object class in Java has three final methods that allow threads to communicate about the locked status of a resource. waitIt tells the calling thread to give up the lock and go to sleep until some other thread enters the same monitor and calls notify.
Java Consumer Producer
The wait method releases the lock prior to waiting and reacquires the lock prior to returning from the wait method. The wait method is actually tightly integrated with the synchronization lock, using a feature not available directly from the synchronization mechanism.In other words, it is not possible for us to implement the wait method purely in Java. It is a native method.General syntax for calling wait method is like this. This is an excellent article. An example could be group of threads (one thread processing only a specific type of message) watching a message queue. Upon placing a message in queue, producer can notify all consumer threads.
Producer Consumer Problem C++
Then each consumer thread may check if message is for it, and if it is then process it otherwise wait again.This design may look inefficient as correct thread may get chance to check in last; BUT it will start making sense when you start adding more message types and their handler threads into system without modifying code of other handlers.Just for an example.